Table of Contents
Toggle“Pick 4 Tic Tac Toe” – Introducing a New Era
The traditional game of ‘Tic Tac Toe’ is making a comeback with a new twist – ‘Pick 4 Tic Tac Toe’. This latest iteration is weaving an indelible thread into the tapestry of children’s formative years, imprinting memories that dance with novelty.
Moreover, it beckons to the realm of strategic contemplation, extending an invitation to aficionados of cerebral engagements. It unfurls a canvas where victory materializes not solely in the alignment of three, but also blooms from the arrangement of four, bestowing a challenge that whispers of uncharted triumphs.
In this article, we tell you into the process of creating the ‘Pick 4 Tic Tac Toe’ game in Python – a game that not only comes with more possibilities but also provides an enhanced gaming experience.
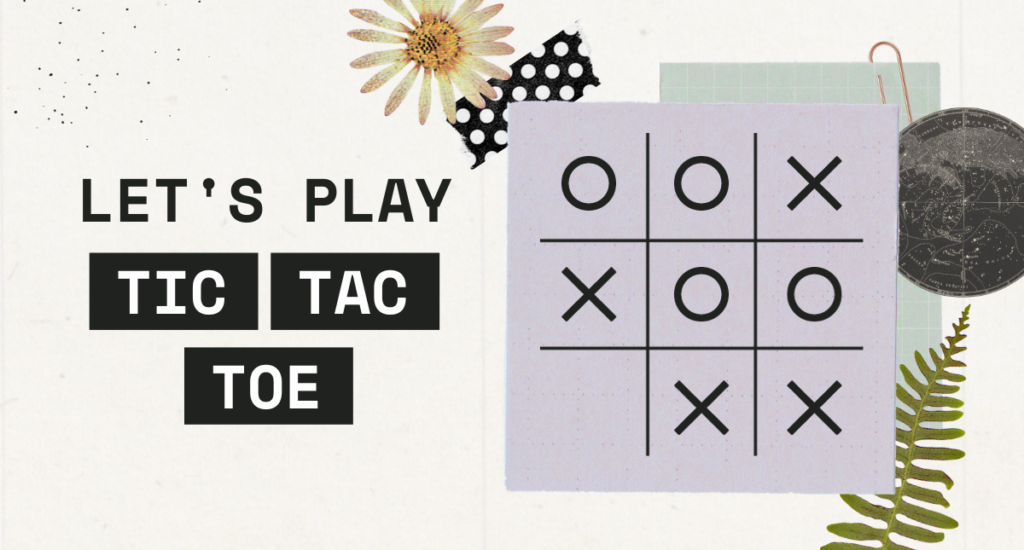
Description of ‘Pick 4 Tic Tac Toe’
The traditional ‘Tic Tac Toe’ is played on a 3×3 grid, where two players mark their symbols (X and O) to form three in a row, column, or diagonal. Now, in ‘Pick 4 Tic Tac Toe’, we’ve expanded the game onto a larger 4×4 grid. Players now need to form a line of four symbols, allowing them to achieve victory. Gradually, this tiny alteration enhances the game’s intricacy and tactics, adding an extra layer of challenge and excitement.
Setting Up the Board for Players
Before diving into the coding process, we need to prepare the game board for the players. We’ve structured the game board as a 2D list, where each element represents a cell on the board. Initially, we create the board with empty cells and allow players to update cells with their symbols. The following code snippet demonstrates how to create the board:
def create_board():
board = [[' ' for _ in range(4)] for _ in range(4)]
return board
Displaying the Board
To visually represent the game board’s status with visual representations of players’ symbols, we need to create a function. This function formats the cells of the board properly and prints players’ symbols based on their choices. The following code snippet shows how to display the board:
def display_board(board):
for row in board:
print('|'.join(row))
print('-' * 9)
Obtaining Input from Players
After preparing the board, we need a mechanism to gather information from players about the positions of their symbols and update the board accordingly. We’ve created a function that prompts players to choose a row and column for their move. The function ensures that the selected cell is empty and updates it based on the player’s symbol. The code snippet below demonstrates how to obtain input from players:
def get_player_move(board, player):
while True:
try:
row, col = map(int, input(f"Player {player}, enter row and column (0-3) separated by a space: ").split())
if 0 <= row < 4 and 0 <= col < 4 and board[row][col] == ' ':
return row, col
else:
print("Invalid move. Please try again.")
except ValueError:
print("Invalid input. Enter two numbers between 0 and 3 separated by a space.")
Checking for a Winner
To check for a player’s victory condition, we’ve created a function that examines rows, columns, and diagonals for a sequence of four symbols. This function will be called after each player’s move. If a player successfully forms four symbols in any direction, the game ends, and the winner is announced. The following code snippet checks for a winner:
def check_winner(board, player, row, col):
directions = [(1, 0), (0, 1), (1, 1), (-1, 1)] # Possible directions for checking
for dr, dc in directions:
count = 0
r, c = row, col
while 0 <= r < 4 and 0 <= c < 4 and board[r][c] == player:
count += 1
r += dr
c += dc
if count == 4:
return True
return False
Playing the Game
Combining all the components, we can create a game loop that keeps running between the players. In this loop, players’ turns will alternate, and the loop will accept and process input, update the board, and check for a winner. The game continues until a player wins or the board is filled:
def play_pick4_tic_tac_toe():
board = create_board()
player = 'X'
for _ in range(16): # Maximum 16 moves in a 4x4 board
display_board(board)
row, col = get_player_move(board, player)
board[row][col] = player
if check_winner(board, player, row, col):
display_board(board)
print(f"Player {player} wins!")
break
player = 'O' if player == 'X' else 'X'
else:
display_board(board)
print("It's a draw!")
Conclusion
In this tutorial, we’ve explained the process of creating the game ‘Pick 4 Tic Tac Toe’ in Python, introducing a new and exciting direction. We’ve already talked about how we show the game board, get what players do, and see who wins using different tools. You can make it even better by adding cool stuff like a computer opponent or making it look prettier with fancy pictures. Enjoy coding and have fun playing ‘Pick 4 Tic Tac Toe’!
Read more articles : Worked in Python or Java, say Accurate NYT Crossword Clue (6 March 2023).
Understanding Post-increment and Pre-increment in C Programming