If you’re looking to add a dynamic touch to your web page, incorporating 3D animations with CSS and HTML can be a great way to capture attention and showcase creativity. In this blog post, we’ll dive into a CSS-based 3D rotation animation that can bring a striking visual element to your site. We’ll break down the CSS and HTML used to create a rotating 3D text effect that can add flair to any page. Let’s explore how this code works and how you can customize it for your own projects.
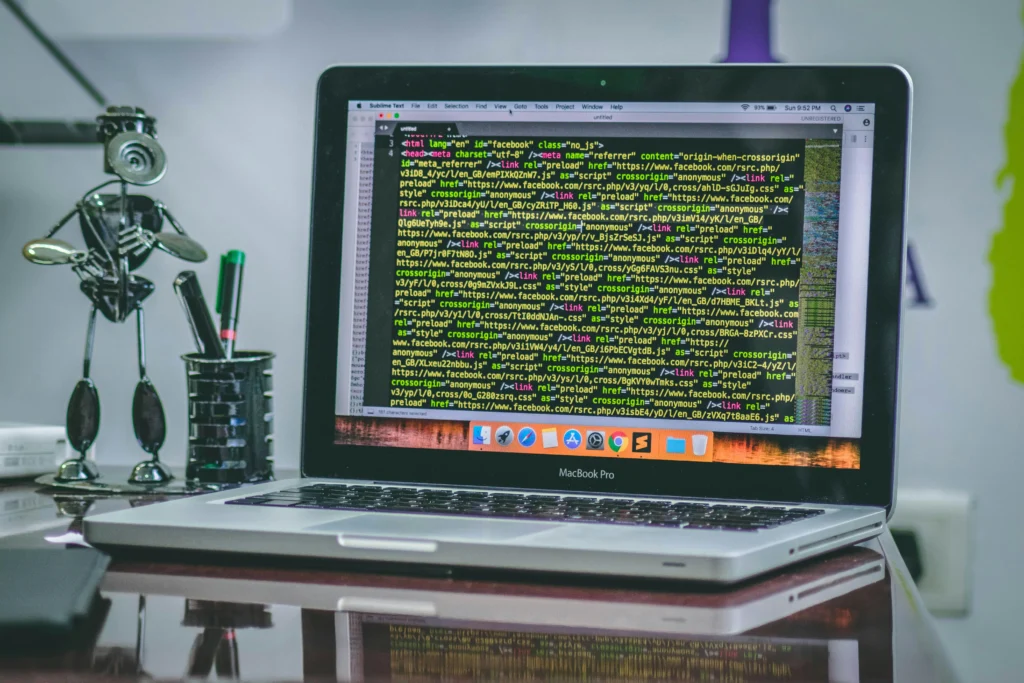
Table of Contents
ToggleThe HTML Structure for 3D rotation using CSS and HTML
First, let’s look at the HTML structure of our animation:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Eat Sleep Rave 3D Rotation</title>
</head>
<body>
<div class="container">
<div class="box">
<span style="--i:1">
<i>EAT</i>
SLEEP
<i>RAVE</i>
</span>
<!-- Repeat the <span> element with different values for --i -->
</div>
</div>
</body>
</html>
In this structure, we have a container div and a box div inside it. The box contains multiple span
elements that hold the text content. Each span
is styled with a custom property (--i
) to create different effects for each rotation layer.
CSS Styling and Animation
Here’s the CSS that brings the 3D effect to life:
@import url('https://fonts.googleapis.com/css2?family=Heebo:wght@400;600&family=Poppins:wght@100;500;600;800;900&family=Lobster+Two:ital,wght@0,400;0,700;1,400&display=swap');
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
select:focus, input:focus, button:focus {
outline: none;
}
body {
overflow: hidden;
background-color: #222;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
min-height: 100vh;
}
.container {
display: flex;
justify-content: center;
align-items: center;
}
.box {
transform-style: preserve-3d;
animation: animate 7s ease-in-out infinite;
}
span {
background: linear-gradient(90deg, rgba(0, 0, 0, 0.1), rgba(0, 0, 0, 0.5) 90%, transparent);
text-transform: uppercase;
line-height: 0.76em;
position: absolute;
color: white;
font-size: 3.5em;
white-space: nowrap;
font-weight: bold;
padding: 0px 10px;
transform-style: preserve-3d;
text-shadow: 0 10px 15px #333;
transform: translate(-50%, -50%) rotateX(calc(var(--i) * 22.5deg)) translateZ(109px);
}
i {
font-style: initial;
}
i:nth-child(1) {
color: #5c5fc4;
}
i:nth-child(2) {
color: #c4c15c;
}
@keyframes animate {
0% {
transform: perspective(500px) rotateX(0deg) rotate(5deg);
}
100% {
transform: perspective(50px) rotateX(360deg) rotate(5deg);
}
}
Key Components
- Global Reset: The
*
selector ensures all elements have consistent padding and margin, which helps in avoiding layout inconsistencies. - Container and Box Styling: The
.box
class is crucial for applying the 3D effect. Thetransform-style: preserve-3d;
property ensures that child elements maintain their 3D transformations. Theanimation
property applies a continuous rotation effect to the.box
element. - Text Styling: Each
span
element represents a different layer in the 3D space. Thetransform
property is used to position each layer and create the rotating effect. TherotateX
transformation is combined withtranslateZ
to move the text along the Z-axis, giving it a 3D appearance. - Animation: The
@keyframes
rule defines the animation, rotating the.box
element along the X-axis, creating a smooth 360-degree rotation effect.
Transformations and Perspective
The transform
property in CSS is key to creating 3D effects. Here’s a deeper look at the specific transformations used in our code:
translate(-50%, -50%)
: This shifts the element to ensure it’s centered properly within its container.rotateX(calc(var(--i) * 22.5deg))
: This rotates each text layer around the X-axis. Thecalc
function allows us to use a custom property (--i
) to control the rotation dynamically. This is what creates the layered effect in the animation.translateZ(109px)
: Moves the text along the Z-axis, pushing it away from the viewer to enhance the 3D effect.
The perspective
property in the keyframes provides a sense of depth by altering how the 3D space is viewed. A smaller perspective value, like 50px
, gives a more pronounced 3D effect compared to a larger value like 500px
.
Keyframes Animation
The @keyframes
rule defines the animation sequence. Here’s a breakdown:
0%
: The animation starts with no rotation (rotateX(0deg)
) and a perspective of500px
, making the text appear less dynamic.100%
: The animation ends with a full rotation (rotateX(360deg)
) and a closer perspective (50px
), giving the text a complete 360-degree turn in 3D space.
This continuous loop (infinite
) ensures that the rotation effect never stops, creating a mesmerizing visual for the viewer.
Customization Tips
- Text Content: Modify the text inside the
span
elements to fit your needs. You can also change the colors and fonts to match your website’s theme. - Animation Speed: Adjust the
7s
in theanimation
property to make the rotation faster or slower. - Perspective: Tweak the
perspective
values in the@keyframes
to change the depth of the rotation.
Conclusion
This 3D rotation animation is a great way to add some eye-catching effects to your web page. By leveraging CSS animations and 3D transforms, you can create dynamic and engaging visual experiences with relatively simple CSS and HTML codes. Experiment with different text, colors, and animation settings to make this effect your own!
Feel free to drop any questions or customizations you’d like to explore in the comments below. Happy coding!