Welcome to our fun and funky blog post where we delve into the details of creating a playful dancing robot using HTML and CSS. Meet Gary, our charming robot who just wants to boogie! In this post, we’ll explore the code that brings Gary to life and how you can tweak it to add your own creative touches.
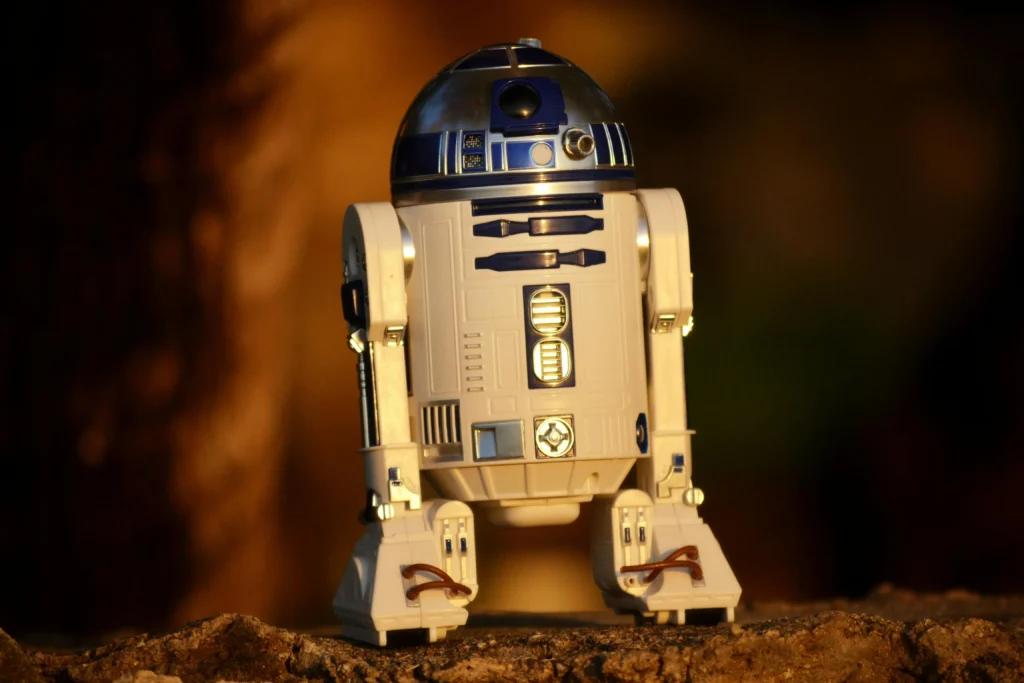
Table of Contents
ToggleThe HTML Structure for Gary robot
Here’s the backbone of our dancing robot:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Help Gary to Dance!</title>
</head>
<body>
<h1>Gary the Dancing Robot</h1><br><br>
<div class="robots">
<div class="android">
<div class="head">
<div class="eyes">
<div class="left_eye"></div>
<div class="right_eye"></div>
</div>
</div>
<div class="upper_body">
<div class="left_arm"></div>
<div class="torso"></div>
<div class="right_arm"></div>
</div>
<div class="lower_body">
<div class="left_leg"></div>
<div class="right_leg"></div>
</div>
</div>
</div>
<div class="Gary">Gary is a little shy. Help him dance with your cursor!</div>
</body>
</html>
The HTML creates a simple structure with a header, a dancing robot section, and a message to encourage interaction.
Styling Gary with CSS
Now, let’s dive into the CSS that brings Gary to life. We’ll explore how each section contributes to the overall dancing effect.
General Styles
h1 {
text-align: center;
font-family: 'Roboto', sans-serif;
border-left: solid 5px #A286FD;
border-right: solid 5px #A286FD;
color: #A286FD;
font-style: italic;
width: 400px;
left: 300px;
margin: auto;
background-color: #CFC0FF;
padding: 10px 10px;
}
body {
background-image: radial-gradient(circle, #A286FD, #CFC0FF, #6E49E6);
}
Here, we center the heading and apply some stylish borders and colors. The body background features a playful radial gradient to set the scene.
Robot Parts
Each part of Gary is styled with CSS, and here’s where the magic happens:
- Head
.head {
width: 200px;
height: 150px;
border-radius: 200px 200px 0 0;
margin: 0 auto 10px auto;
background-color: #F5387E;
background-image: url("https://wallpaperaccess.com/full/31367.jpg");
}
.head:hover {
width: 225px;
transition: .5s ease-in-out;
animation: headbop 2s infinite;
box-shadow: 0px 0px 15px #CFC0FF;
}
@keyframes headbop {
0% {transform: translate(0px, 0px)}
25% {transform: translate(0px, 10px)}
50% {transform: translate(0px, 0px)}
75% {transform: translate(0px, 10px)}
100% {transform: translate(0px, 0px)}
}
The head has a bouncing animation that activates on hover, thanks to the headbop
keyframes. This effect makes Gary’s head move up and down, giving him a lively appearance.
- Arms
.left_arm, .right_arm {
width: 40px;
height: 125px;
border-radius: 100px;
transition: transform .5s;
background-color: #F5387E;
}
.left_arm:hover {
transform: rotate(20deg);
box-shadow: 0px 0px 15px #CFC0FF;
animation: la-wiggle 2s infinite;
}
.right_arm:hover {
transform: rotate(-20deg);
box-shadow: 0px 0px 15px #CFC0FF;
animation: ra-wiggle 2s infinite;
}
@keyframes la-wiggle {
0% {transform: rotate(20deg);}
25% {transform: rotate(0deg);}
50% {transform: rotate(20deg);}
75% {transform: rotate(0deg);}
100% {transform: rotate(20deg);}
}
@keyframes ra-wiggle {
0% {transform: rotate(-20deg);}
25% {transform: rotate(0deg);}
50% {transform: rotate(-20deg);}
75% {transform: rotate(0deg);}
100% {transform: rotate(-20deg);}
}
The arms have a wiggling effect when hovered over. Each arm rotates back and forth, simulating dancing moves.
- Torso
.torso {
width: 200px;
height: 200px;
border-radius: 0 0 50px 50px;
transition: transform .5s;
background-color: #F5387E;
}
.torso:hover {
transform: translate(0px, 10px);
animation: wiggle 2s infinite;
box-shadow: 0px 0px 15px #CFC0FF;
}
@keyframes wiggle {
0% {transform: translate(0px, 0px)}
25% {transform: translate(10px, 0px)}
50% {transform: translate(0px, 0px)}
75% {transform: translate(-10px, 0px)}
100% {transform: translate(0px, 0px)}
}
The torso wiggles side to side, adding to Gary’s dancing dynamism.
- Legs
.left_leg, .right_leg {
width: 40px;
height: 120px;
border-radius: 0 0 100px 100px;
transition: transform .5s;
background-color: #F5387E;
}
.left_leg:hover {
transform: rotate(20deg);
box-shadow: 0px 0px 15px #CFC0FF;
animation: ll-wiggle 2s infinite;
}
.right_leg:hover {
transform: rotate(-20deg);
box-shadow: 0px 0px 15px #CFC0FF;
animation: rl-wiggle 2s infinite;
}
@keyframes ll-wiggle {
0% {transform: rotate(20deg);}
25% {transform: rotate(0deg);}
50% {transform: rotate(20deg);}
75% {transform: rotate(0deg);}
100% {transform: rotate(20deg);}
}
@keyframes rl-wiggle {
0% {transform: rotate(-20deg);}
25% {transform: rotate(0deg);}
50% {transform: rotate(-20deg);}
75% {transform: rotate(0deg);}
100% {transform: rotate(-20deg);}
}
The legs rotate in opposite directions when hovered over, adding to the dancing effect. The `ll-wiggle` and `rl-wiggle` keyframes create a playful dance routine for Gary’s legs.
- **Eyes**
```css
.left_eye, .right_eye {
width: 20px;
height: 20px;
border-radius: 15px;
background-color: #A286FD;
}
.left_eye {
position: relative;
top: 100px;
left: 40px;
}
.right_eye {
position: relative;
top: 100px;
left: 120px;
}
The eyes are small, circular, and positioned to give Gary a friendly appearance. They don’t animate, but their placement helps in defining Gary’s expression.
The Gary Message
.Gary {
text-align: center;
font-family: 'Roboto', sans-serif;
color: #A286FD;
font-weight: bold;
font-size: 1.5em;
background-color: #CFC0FF;
padding: 10px 10px;
width: 400px;
margin: auto;
border-left: solid 5px #A286FD;
border-right: solid 5px #A286FD;
}
This section provides a fun and friendly message to users, encouraging them to interact with Gary and help him dance. The styling matches the theme of the rest of the page, making it visually cohesive.
Conclusion
Gary the Dancing Robot is a fun project that combines basic HTML structure with dynamic CSS animations. By hovering over different parts of Gary, users can see him dance and interact with the page. This simple yet engaging code demonstrates the power of CSS animations and how they can be used to create interactive web experiences.
Feel free to experiment with the CSS properties and animations to make Gary even more lively or to add your own personal touch to this dancing robot. Happy coding, and may your robots always dance with joy!