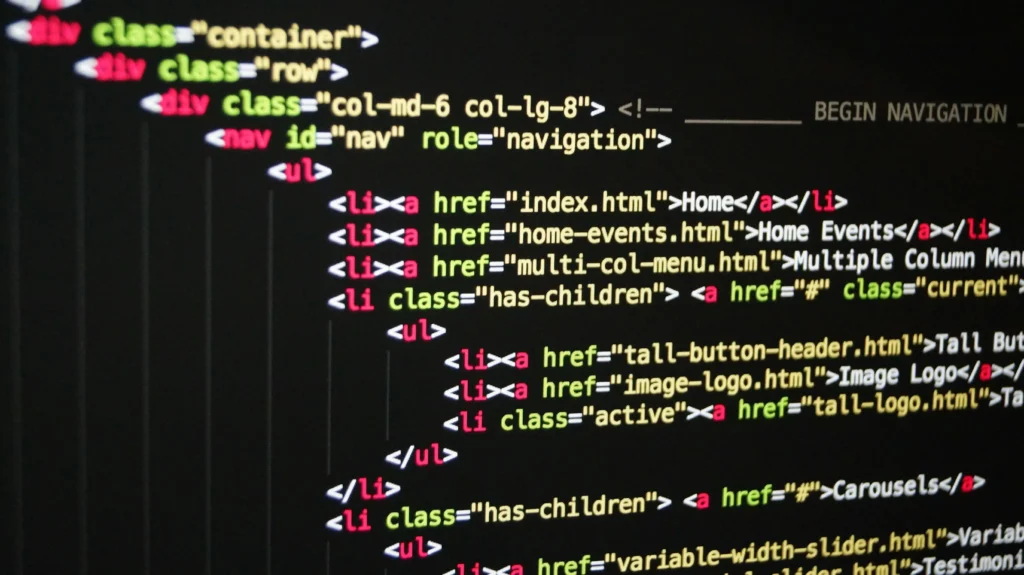
In the world of web design and development, CSS animations offer a powerful tool to add captivating visual effects to your websites. In this blog post, we’ll dissect an interesting example of CSS animation: a bouncing bowl with a dynamically rotating liquid effect. The code provided demonstrates a blend of CSS properties and keyframe animations that bring a bouncing bowl to life on the web page. Let’s dive into the details and understand how each part contributes to the overall effect.
Table of Contents
ToggleHTML Structure of bouncing bowl animation
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
/* CSS code goes here */
</style>
<body>
<section>
<div class="shadow"></div>
<div class="bowl">
<div class="liquid"></div>
</div>
</section>
</body>
</html>
In this HTML structure, we have a simple layout with a <section>
containing three main elements:
shadow
: Represents the shadow of the bouncing bowl.bowl
: The main container for the animated bowl.liquid
: The element inside the bowl that simulates the liquid.
CSS Breakdown
The CSS styles and animations are where the magic happens. Let’s break down each part.
Global Styles and Section Layout
@import url('https://fonts.googleapis.com/css2?family=BhuTuka+Expanded+One&family=Kanit&family=Mrs+Sheppards&family=Oswald:wght@700&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
section {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
background: #121212;
}
- The
@import
rule is used to bring in Google Fonts, though they aren’t used in the visible styles. - The
*
selector removes default margin and padding and setsbox-sizing
toborder-box
for a more predictable box model. - The
section
styles center the content both horizontally and vertically and set a dark background color.
The Animated Bowl
.bowl {
position: relative;
width: 300px;
height: 300px;
background: rgba(255, 255, 255, 0.1);
border-radius: 50%;
border: 8px solid transparent;
animation: animate 5s linear infinite;
transform-origin: bottom center;
animation-play-state: run;
}
@keyframes animate {
0% {
transform: rotate(0deg);
}
25% {
transform: rotate(22deg);
}
50% {
transform: rotate(0deg);
}
75% {
transform: rotate(-22deg);
}
100% {
filter: hue-rotate(360deg);
}
}
- The
.bowl
class creates a circular element with a semi-transparent background and a border. It uses therotate
transform to create a wobbling effect. - The
@keyframes animate
rule defines the animation sequence, rotating the bowl back and forth and applying a hue rotation filter to create a color-changing effect.
Bowl Details and Shadows
.bowl::before {
content: '';
position: absolute;
top: -15px;
left: 50%;
transform: translateX(-50%);
width: 40%;
height: 30px;
border: 15px solid #444;
border-radius: 50%;
box-shadow: 0 10px #222;
}
.bowl::after {
content: '';
position: absolute;
top: 35%;
left: 50%;
transform: translate(-50%, -50%);
border-radius: 50%;
width: 150px;
height: 60px;
background: rgba(255, 255, 255, 0.05);
transform-origin: center;
animation: animateBowlShadow 5s linear infinite;
}
@keyframes animateBowlShadow {
0% {
left: 50%;
width: 150px;
height: 60px;
}
25% {
left: 55%;
width: 140px;
height: 65px;
}
50% {
left: 50%;
width: 150px;
height: 60px;
}
75% {
left: 45%;
width: 140px;
height: 65px;
}
}
- The
::before
pseudo-element creates the bowl’s rim with a shadow to give it depth. - The
::after
pseudo-element simulates the shadow of the bowl with an animation that makes it pulse and move.
The Liquid Animation
.liquid {
position: absolute;
top: 50%;
left: 5px;
right: 5px;
bottom: 5px;
background: #1ae907;
border-bottom-left-radius: 150px;
border-bottom-right-radius: 150px;
filter: drop-shadow(0 0 80px #1ae907);
transform-origin: top center;
animation: animateLiquid 5s linear infinite;
}
@keyframes animateLiquid {
0% {
transform: rotate(0deg);
}
25% {
transform: rotate(-22deg);
}
30% {
transform: rotate(-23deg);
}
50% {
transform: rotate(0deg);
}
75% {
transform: rotate(22deg);
}
80% {
transform: rotate(23deg);
}
100% {
transform: rotate(0deg);
}
}
.liquid::before {
content: '';
position: absolute;
top: -10px;
width: 100%;
height: 20px;
background: #15be05;
border-radius: 50%;
filter: drop-shadow(0 0 80px #15be05);
}
- The
.liquid
class styles the liquid inside the bowl with a bright green color and an animated rotation effect. - The
::before
pseudo-element adds a highlight to the liquid, enhancing its visual appeal.
Shadow Animation
.shadow {
position: absolute;
top: calc(50% + 150px);
left: 50%;
transform: translate(-50%, -50%);
width: 260px;
height: 40px;
background: rgba(0, 0, 0, 0.5);
border-radius: 50%;
animation: animteShadow 5s linear infinite;
}
@keyframes animteShadow {
0% {
left: 50%;
width: 260px;
height: 40px;
top: calc(50% + 150px);
}
25% {
left: 55%;
width: 265px;
height: 30px;
top: calc(50% + 130px);
}
50% {
left: 50%;
width: 260px;
height: 40px;
top: calc(50% + 150px);
}
75% {
left: 45%;
width: 265px;
height: 30px;
top: calc(50% + 130px);
}
}
- The
.shadow
class creates a shadow that animates to mimic the motion of the bowl, adding realism to the overall effect.
Conclusion
This code showcases how CSS animations can be used creatively to simulate complex effects like a bouncing bowl with liquid. By combining @keyframes
with CSS properties such as transform
, filter
, and box-shadow
, you can craft engaging visual experiences that enhance your web pages. Understanding these principles not only helps in creating animations but also in debugging and optimizing them for better performance and user experience.
Feel free to experiment with different properties and animations to make your designs even more dynamic and visually appealing. Happy coding!