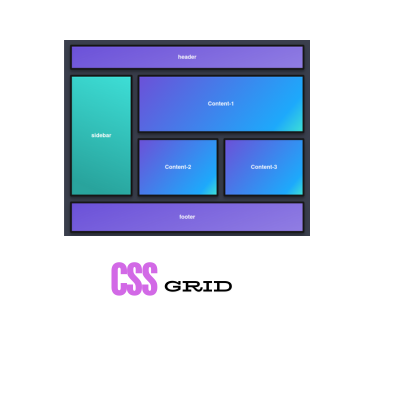
One of CSS’s most potent layout systems is CSS Grid Layout. It makes it simple for developers to design intricate and responsive site layouts. Here is a quick introduction to CSS Grid along with some sample code.
Table of Contents
ToggleBasic Concepts
CSS Grid Container
The component that has the grid display applied to it. It turns into a grid container, and the grid elements are its offspring.
//CSS
.container {
display: grid; /* or display: inline-grid; */
}
CSS Grid Items
The grid container’s direct offspring.
The direct offspring from the grid container are called grid objects. These components are arranged inside the predetermined grid.
//Html
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
</div>
CSS Grid Lines
The horizontal and vertical lines divide the grid.
The vertical and horizontal lines that separate the grid container through cells are called grid lines. Grid elements are positioned and sized using them.
1. Grid lines that are horizontal run from left to right.
2. The grid’s vertical lines extend from top to bottom.
At the grid’s boundaries, numbers for the grid lines begin at 1.
CSS Grid Tracks
The columns, as well as rows of the grid, are called grid tracks. These are the areas that separate two neighboring grid lines.
1. Grid Row: The distance on a horizontal grid line between two lines.
2. Grid Column: The distance in an orthogonal grid between two lines.
//CSS
.container {
display: grid;
grid-template-columns: 100px 200px 100px; /* 3 columns */
grid-template-rows: 50px 100px; /* 2 rows */
}
CSS Grid Cells
The smallest unit on the grid, a grid cell is determined by the point where a row and a column intersect.
CSS Grid Areas
The start row, end row, start column, and end column are the four grid lines that define the rectangular grid areas. Grid items can be placed into and named within these sections.
//CSS
.container {
display: grid;
grid-template-areas:
"header header header"
"menu main main"
"footer footer footer";
}
.header {
grid-area: header;
}
.menu {
grid-area: menu;
}
.main {
grid-area: main;
}
.footer {
grid-area: footer;
}
Practical Example
Using the ideas discussed above, let’s construct a simple example:
//CSS
.container {
display: grid;
grid-template-columns: 1fr 2fr;
grid-template-rows: auto 1fr auto;
grid-template-areas:
"header header"
"menu main"
"footer footer";
gap: 10px;
}
.header {
grid-area: header;
background-color: lightblue;
}
.menu {
grid-area: menu;
background-color: lightcoral;
}
.main {
grid-area: main;
background-color: lightgreen;
}
.footer {
grid-area: footer;
background-color: lightgrey;
}
In this instance:
- The two columns and three rows of the layout are defined by the CSS grid container.
- Named areas in the grid are specified by the grid-template-areas attribute.
- Grid items are assigned to the designated areas via the grid-area attribute.
Conclusion
Gaining an understanding of these fundamental ideas will enable you to fully utilize CSS Grid Layout. You may effectively construct flexible and responsive web designs by becoming proficient with grid containers, grid items, grid lines, grid tracks, grid cells, & grid regions. Try varying these attributes to observe how they affect each other and your layout.
Basic Properties
Display
The element is defined as a container for a CSS grid.
//CSS
.container {
display: grid; /* or display: inline-grid; */
}
Grid-template-columns
and Grid-template-rows
Describes the grid’s rows and columns. Values such as px, %, fr (fractional unit), auto, as well as others are available for use.
//CSS
.container {
display: grid;
grid-template-columns: 100px 200px 1fr; /* Three columns */
grid-template-rows: 50px auto; /* Two rows */
}
CSS Grid-template-areas
Specifies the grid container’s named areas. Utilize this in addition to grid items’ grid-area property.
//CSS
.container {
display: grid;
grid-template-areas:
"header header header"
"menu main main"
"footer footer footer";
}
.header { grid-area: header; }
.menu { grid-area: menu; }
.main { grid-area: main; }
.footer { grid-area: footer; }
Grid-column-gap
, Grid-row-gap
, and Gap
Establishes the distance between rows and columns.
//CSS
.container {
display: grid;
grid-template-columns: 1fr 1fr;
grid-template-rows: 1fr 1fr;
grid-column-gap: 10px; /* Gap between columns */
grid-row-gap: 15px; /* Gap between rows */
gap: 10px 15px; /* Shorthand for grid-column-gap and grid-row-gap */
}
Grid-column
and Grid-row
Specifies where the grid items’ beginning and ending lines should be.
//CSS
.item1 {
grid-column: 1 / 3; /* Start at column line 1, end before column line 3 */
grid-row: 1 / 2; /* Start at row line 1, end before row line 2 */
}
Grid-area
Shorthand is used to assign objects to certain grid regions or to specify grid-row-start, grid-column-start, grid-row-end, & grid-column-end.
//CSS
.item1 {
grid-area: 1 / 2 / 3 / 4; /* row-start / column-start / row-end / column-end */
}
Alternatively, using designated grid areas:
//CSS
.item1 {
grid-area: header; /* Assigns the item to the 'header' area */
}
Justify-items
, Align-items
, Justify-content
, and Align-content
Grid elements are aligned concerning the grid container and their cells.
//CSS
.container {
display: grid;
justify-items: center; /* Aligns items horizontally within their cells */
align-items: center; /* Aligns items vertically within their cells */
justify-content: center; /* Aligns the grid container horizontally */
align-content: center; /* Aligns the grid container vertically */
}
These characteristics have start, end, center, & stretch as possible values.
Grid-auto-columns
, Grid-auto-rows
, and Grid-auto-flow
specifies the location of automatically put items and the size for implicitly generated grid tracks.
//CSS
.container {
display: grid;
grid-auto-columns: 100px; /* Size for implicit columns */
grid-auto-rows: 50px; /* Size for implicit rows */
grid-auto-flow: row; /* Controls auto-placement algorithm */
}
Practical Example
Now let’s use some of these more sophisticated features to create a more complicated example.
HTML
<div class="container">
<div class="header">Header</div>
<div class="menu">Menu</div>
<div class="main">Main</div>
<div class="footer">Footer</div>
</div>
CSS
.container {
display: grid;
grid-template-columns: 1fr 3fr;
grid-template-rows: auto 1fr auto;
grid-template-areas:
"header header"
"menu main"
"footer footer";
gap: 10px;
justify-items: stretch;
align-items: stretch;
}
.header {
grid-area: header;
background-color: lightblue;
}
.menu {
grid-area: menu;
background-color: lightcoral;
}
.main {
grid-area: main;
background-color: lightgreen;
}
.footer {
grid-area: footer;
background-color: lightgrey;
}
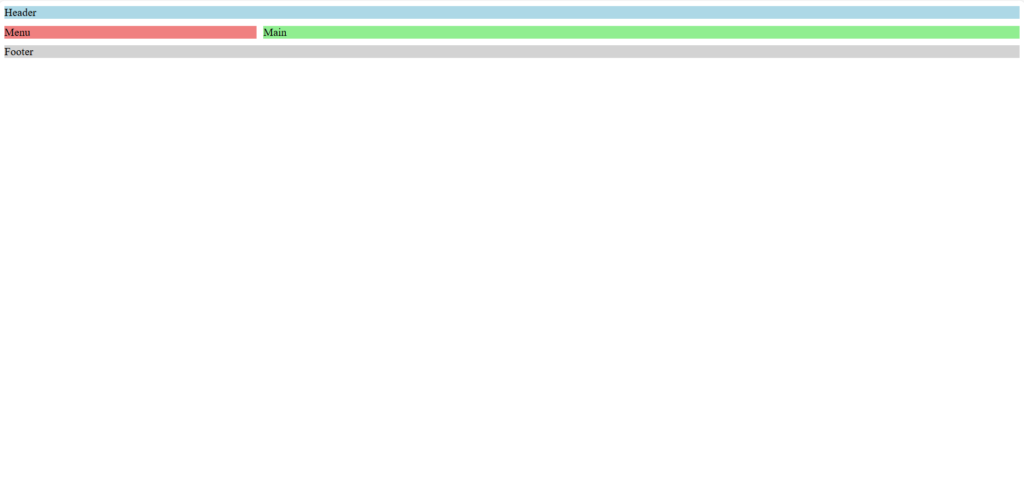
Conclusion
You can effectively establish and control the arrangement for your grid containers & items by becoming proficient with these fundamental attributes. Try varying these attributes to observe their impact on the grid and develop adaptable, responsive web designs.
Advanced Features
Named Grid Lines
To make item placement more readable and manageable, you can give grid line names.
//CSS
.container {
display: grid;
grid-template-columns: [start] 100px [middle] 200px [end];
grid-template-rows: [row1-start] 100px [row1-end row2-start] 100px [row2-end];
}
.item1 {
grid-column: start / middle;
grid-row: row1-start / row1-end;
}
Grid Template Areas with Span
An object can span numerous rows or columns by using the span keyword.
//CSS
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-template-rows: repeat(2, 1fr);
}
.item1 {
grid-column: 1 / span 2; /* Span 2 columns */
grid-row: 1 / span 2; /* Span 2 rows */
}
Fractional Units (fr
)
A small portion of the grid container’s available space is allotted by the fr unit.
//CSS
.container {
display: grid;
grid-template-columns: 1fr 2fr 1fr; /* 1 part, 2 parts, 1 part */
}
Subgrid
A grid item can inherit its grid definition from the original grid container thanks to the subgrid capability. Note: Because of my most recent version, the subgrid is only partially supported by other browsers and exclusively by Firefox.
//CSS
.container {
display: grid;
grid-template-columns: 1fr 1fr;
grid-template-rows: 1fr 1fr;
}
.subcontainer {
display: subgrid;
grid-column: 1 / 3;
grid-template-rows: subgrid;
}
Aligning and Justifying Grid Items Individually
By utilizing justify-self and align-self, you may align and justify each grid item separately.
//CSS
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 1fr 1fr;
}
.item1 {
justify-self: center; /* Center horizontally */
align-self: end; /* Align to the end vertically */
}
Order Property
Without altering the HTML order of the grid components, you can adjust their visual arrangement using the order property.
//CSS
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 1fr 1fr;
}
.item1 {
order: 2; /* This item will appear after items with lower order values */
}
.item2 {
order: 1; /* This item will appear before items with higher order values */
}
Practical Example
Let’s combine these sophisticated characteristics to produce a more intricate example.
//Html
<div class="container">
<div class="header">Header</div>
<div class="menu">Menu</div>
<div class="main">Main</div>
<div class="sidebar">Sidebar</div>
<div class="footer">Footer</div>
</div>
//CSS
.container {
display: grid;
grid-template-columns: [col1-start] 1fr [col2-start] 2fr [col3-start] 1fr [col3-end];
grid-template-rows: [row1-start] auto [row2-start] 1fr [row3-start] auto [row3-end];
gap: 10px;
}
.header {
grid-column: col1-start / col3-end;
grid-row: row1-start / row2-start;
background-color: lightblue;
}
.menu {
grid-column: col1-start / col2-start;
grid-row: row2-start / row3-start;
background-color: lightcoral;
}
.main {
grid-column: col2-start / col3-start;
grid-row: row2-start / row3-start;
background-color: lightgreen;
}
.sidebar {
grid-column: col3-start / col3-end;
grid-row: row2-start / span 2;
background-color: lightyellow;
}
.footer {
grid-column: col1-start / col3-end;
grid-row: row3-start / row3-end;
background-color: lightgrey;
}
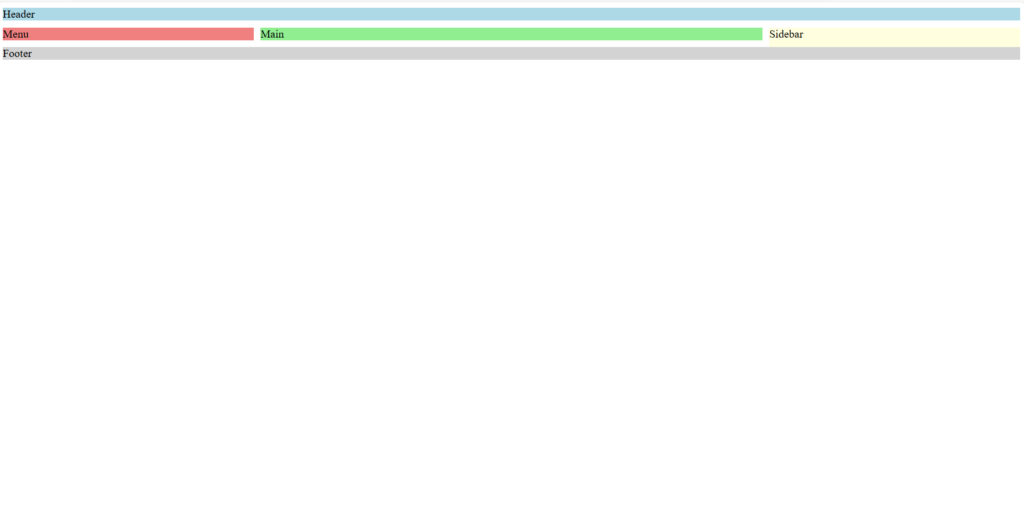
Explanation
- Named Grid Lines: To make reference easier, the grid’s rows and columns have names.
- Span: There are two rows on the sidebar.
- Fractional Units (fr): A proportionate way to assign space.
- Order: The order attribute allows you to reorder objects without modifying the HTML structure, even if it isn’t utilized in this example.
Conclusion
Through the utilization of CSS Grid Layout’s extensive features, you may produce more intricate and responsive web designs. You can create much more intricate grid-based layouts if you comprehend and make use of named grid lines, span, fractional units, subgrids, individual alignment, and the order attribute. Try out these attributes and discover how they can help your site design endeavors.
Responsive Design
With CSS Grid Layout, you can create responsive designs by utilizing a variety of approaches to make sure your layout adjusts to multiple screen sizes & devices. Here are some key techniques and examples to assist you in creating responsive grid layouts.
Media Queries
With media queries, you can apply several styles according to the device’s specifications, like screen width.
//CSS
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
gap: 10px;
}
@media (max-width: 800px) {
.container {
grid-template-columns: 1fr 1fr;
}
}
@media (max-width: 500px) {
.container {
grid-template-columns: 1fr;
}
}
Flexible Units (fr
, percentages)
Your grid items can adjust in size about their container if you use flexible units such as fr & percentages.
//CSS
.container {
display: grid;
grid-template-columns: 1fr 2fr;
gap: 10px;
}
Auto-fill and Auto-fit
Using the auto-fill and auto-fit features of the repeat() method, the grid layout can be dynamically adjusted to fit the available space.
- Auto-fill: Regardless of how many columns are vacant, the row is filled with as many as possible.
- Auto-fit: Collapses any empty columns as it fits the columns into the available space.
//CSS
.container {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(150px, 1fr));
gap: 10px;
}
Minmax Function
Grid tracks can be made to adjust to various screen sizes by using the minmax() function, which specifies a range of sizes.
//CSS
.container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(100px, 1fr));
gap: 10px;
}
Template Area Adjustments
Media queries can be used to modify grid template sections for various device layouts.
//CSS
.container {
display: grid;
grid-template-areas:
"header header"
"menu main"
"footer footer";
gap: 10px;
}
@media (max-width: 800px) {
.container {
grid-template-areas:
"header"
"menu"
"main"
"footer";
}
}
Practical Example
Let’s combine these methods to create a responsive grid layout.
//Html
<div class="container">
<div class="header">Header</div>
<div class="menu">Menu</div>
<div class="main">Main</div>
<div class="sidebar">Sidebar</div>
<div class="footer">Footer</div>
</div>
//CSS
/* Base styles */
.container {
display: grid;
grid-template-columns: 1fr 3fr;
grid-template-rows: auto 1fr auto;
grid-template-areas:
"header header"
"menu main"
"footer footer";
gap: 10px;
}
.header {
grid-area: header;
background-color: lightblue;
}
.menu {
grid-area: menu;
background-color: lightcoral;
}
.main {
grid-area: main;
background-color: lightgreen;
}
.sidebar {
grid-area: sidebar;
background-color: lightyellow;
}
.footer {
grid-area: footer;
background-color: lightgrey;
}
/* Responsive styles */
@media (max-width: 800px) {
.container {
grid-template-columns: 1fr;
grid-template-rows: auto auto 1fr auto auto;
grid-template-areas:
"header"
"menu"
"main"
"sidebar"
"footer";
}
}
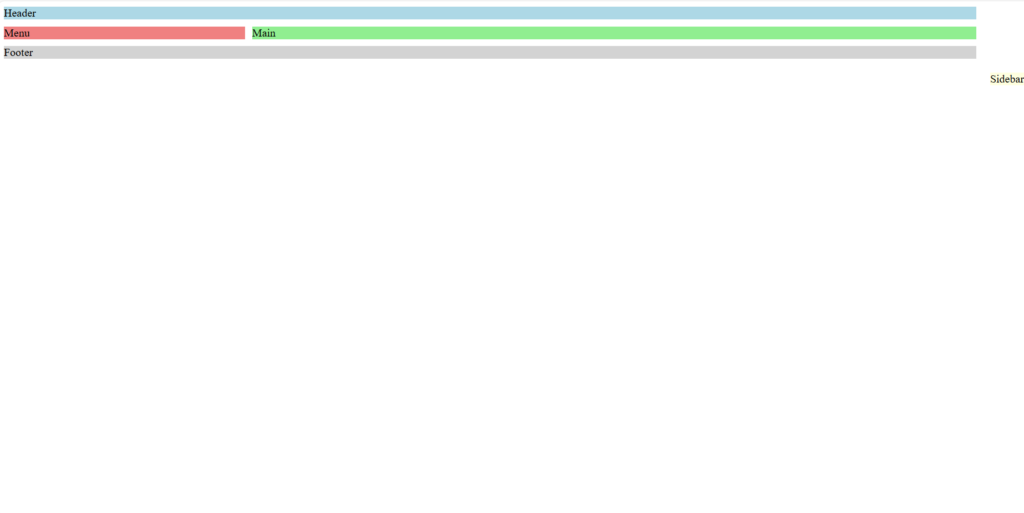
Explanation
- Base Styles: Specify a header, menu, main content, sidebar, & footer area for a two-column layout.
- Responsive styles: To adapt the grid layout for smaller screens, use media queries. The arrangement changes to a single-column format on displays that are smaller than 800 pixels.
Conclusion
Using CSS Grid Layout, media queries, flexible units, auto-fill, auto-fit, minmax, & modifying template sections are necessary to create responsive designs. By using these strategies, you can make sure that your layout adjusts fluidly to various screen sizes & devices, improving user experience. Try out these techniques to make responsive, adaptable web designs.
Conclusion
A powerful tool with lots of functionality for building intricate and responsive web layouts is CSS Grid Layout. You may write little code to construct flexible and effective layouts by familiarizing yourself with its fundamental ideas and characteristics. Try out various attributes and values to observe their impact on the grid and customize your layouts to meet your needs.