Strong techniques for styling and adjusting CSS pictures for a webpage are provided by Cascading Style Sheets or CSS. Some common CSS elements and techniques for working with images are as follows:
Table of Contents
ToggleBasic Image Styling
Go over some basic photo styling techniques in HTML and CSS. Image styling can be used to improve your web pages’ visual appeal and user experience.
Basic HTML for Images
A photo needs to be included in your HTML first. Take note of this basic example:Observe this simple illustration:
//Html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Styling</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<img src="cat.jpg" alt="Description of image" class="style-image">
</body>
</html>
Basic CSS for Image Styling
Let’s now apply a few fundamental styles to the image using your styles.CSS document in CSS.
Setting Width and Height
You can adjust the size of the image by adjusting its width and height.
//CSS
.style-image {
width: 300px;
height: auto; /* Maintains the aspect ratio */
}
Adding Borders
To enclose your image, use borders.
//CSS
.styled-image {
border: 2px solid #000; /* Black border */
border-radius: 10px; /* Rounded corners */
}
Applying Shadows
Box shadows may give your artwork a 3D appearance.
//CSS
.styled-image {
box-shadow: 5px 5px 15px rgba(0, 0, 0, 0.3); /* X offset, Y offset, blur radius, color */
}
Adding Margins and Padding
Control the space around your photo.
//CSS
.styled-image {
margin: 20px; /* Space outside the image / padding: 10px; / Space inside the border */
}
Aligning Images
Pictures can be placed inside their own containers.
//CSS
.styled-image {
display: block; /* Ensures block-level behavior */
margin-left: auto;
margin-right: auto; /* Centers the image horizontally */
}
Responsive Images
Make sure your photographs are responsive so they fit different screen widths.
//CSS
.styled-image {
width: 100%;
max-width: 600px; /* Ensures the image doesn't get too large */
height: auto; /* Maintains aspect ratio */
}
Putting It All Together
Your full CSS file might look like this:
//CSS
.styled-image {
width: 100%;
max-width: 600px;
height: auto;
border: 2px solid #000;
border-radius: 10px;
box-shadow: 5px 5px 15px rgba(0, 0, 0, 0.3);
margin: 20px;
padding: 10px;
display: block;
margin-left: auto;
margin-right: auto;
}
Example Output
Your image will center itself on the page and scale responsively using a border & shadow when you use HTML and CSS to load into your browser.
You can experiment with different values and styles to achieve the desired effect for your pictures.
Rounded Corners
Now let’s focus on using CSS to give photographs rounded borders. Use the border-radius property to do this.
Basic Example with Rounded Corners
Here’s a simple example of how to add rounded edges to an image:
//HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Rounded Corners</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<img src="path-to-your-image.jpg" alt="Description of the image" class="rounded-image">
</body>
</html>
You may create rounded edges on your styles.css file using the border-radius parameter.
//CSS
.rounded-image {
width: 300px;
height: auto; /* Maintain aspect ratio */
border-radius: 15px; /* Adjust the radius to get the desired roundness */
}
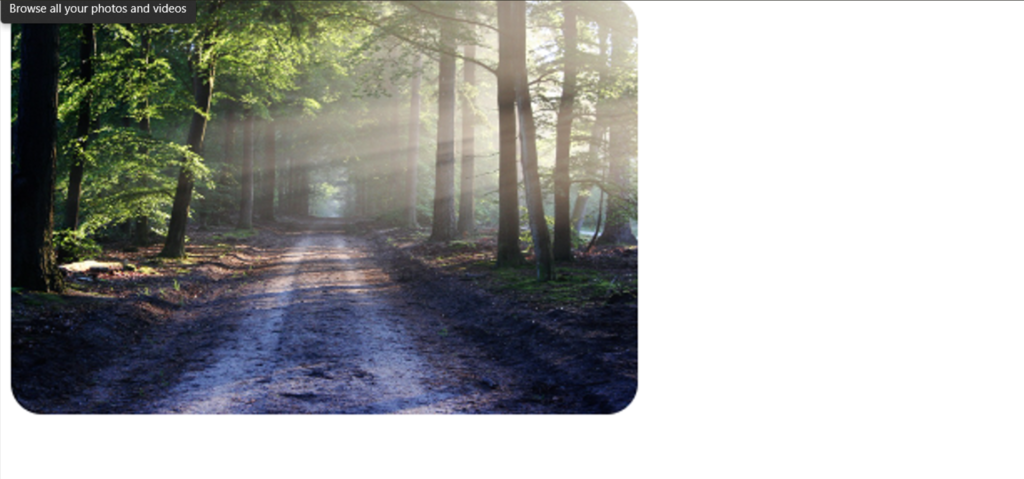
More Examples
Fully Rounded (Circular) Image
Set its border-radius to 50% to create a fully circular image. With square photos, this works best.
//CSS
.rounded-image {
width: 150px;
height: 150px; /* Ensuring the image is square */
border-radius: 50%;
}
Slightly Rounded Corners
You can use a radius that is smaller for somewhat rounded corners.
//CSS
.rounded-image {
width: 300px;
height: auto;
border-radius: 5px;
}
Different Radii for Different Corners
It is also possible to assign distinct radii to every corner.
//CSS
.rounded-image {
width: 300px;
height: auto;
border-radius: 20px 40px 30px 80px; /* Top-left, top-right, bottom-right, bottom-left */
}
Combining Rounded Corners with Other Styles
To improve the appearance, you can pair rounded corners using other designs like borders and shadows.
//CSS
.rounded-image {
width: 300px;
height: auto;
border-radius: 15px;
border: 2px solid #000; /* Border with black color */
box-shadow: 5px 5px 15px rgba(0, 0, 0, 0.3); /* Adding a shadow */
margin: 20px; /* Space around the image */
padding: 10px; /* Space inside the border */
display: block; /* Ensures block-level behavior */
margin-left: auto;
margin-right: auto; /* Centers the image horizontally */
}
Example Output
An image having rounded corners, borders, and a shadow will appear when your browser loads this HTML and CSS. The picture will remain in its original aspect ratio and be centered for the page.
Responsive Rounded Images
You may also render the image responsive to ensure that the rounded corners display effectively on a variety of screen sizes:
//CSS
.rounded-image {
width: 100%;
max-width: 600px; /* Ensures the image doesn't get too large */
height: auto; /* Maintains aspect ratio */
border-radius: 15px;
border: 2px solid #000;
box-shadow: 5px 5px 15px rgba(0, 0, 0, 0.3);
margin: 20px;
padding: 10px;
display: block;
margin-left: auto;
margin-right: auto;
}
This guarantees that the image retains its rounded corners as well as additional aesthetic elements across a range of screen sizes.
To get the desired look, play around with the border-radius and other property values.
Circular Image
Setting that border-radius property for 50% and making sure the picture’s width and height have the same value are necessary steps in using CSS to create a circular image. Here’s a thorough illustration:
HTML and CSS for a Circular Image
HTML Structure:
//HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Circular Image Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="circular-image">
</div>
</body>
</html>
CSS Styling:
//CSS
/* styles.css */
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.circular-image {
width: 250px; /* Set the width */
height: 250px; /* Set the height to the same value as the width */
border-radius: 50%; /* Make the image circular */
object-fit: cover; /* Ensure the image covers the entire area */
border: 2px solid #000; /* Optional: Add a border around the image */
}
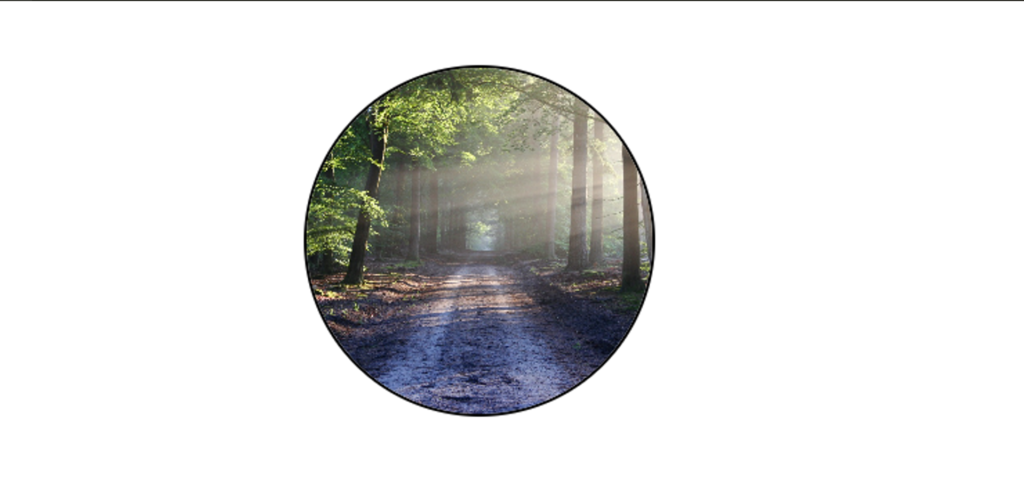
Explanation:
HTML Structure:
- A container div with an IMG tag that the class circular-image makes up the HTML.
- If necessary, the container aids in centering the image both horizontally and vertically.
CSS Styling:
- picture-container centers the picture in the viewport by using Flexbox.
- round-image
.> Width and height: Verify that the picture is square, which is required for circular cropping.
.> border-radius: 50%: Creates a perfect circle in the image by rounding the corners.
.> object-fit: cover: Assures that the picture fits inside the circle frame at the proper scale.
.> border: To improve visual separation, add a custom border around the image.
Full Example:
This is the entire code for an example of a circular image.
//HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Circular Image Example</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.circular-image {
width: 200px; /* Set the width */
height: 200px; /* Set the height to the same value as the width */
border-radius: 50%; /* Make the image circular */
object-fit: cover; /* Ensure the image covers the entire area */
border: 2px solid #000; /* Optional: Add a border around the image */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="circular-image">
</div>
</body>
</html>
With this code, a circular picture centered on the viewport will be produced.
Adding a Border
A circular image might look better and stand out more if it has a border. This is how you use CSS to give a circular image a border.
HTML and CSS for a Circular Image with Border
HTML Structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Circular Image with Border Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="circular-image">
</div>
</body>
</html>
CSS Styling:
/* styles.css */
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.circular-image {
width: 250px; /* Set the width */
height: 250px; /* Set the height to the same value as the width */
border-radius: 50%; /* Make the image circular */
object-fit: cover; /* Ensure the image covers the entire area */
border: 5px solid #000; /* Add a black border around the image */
}
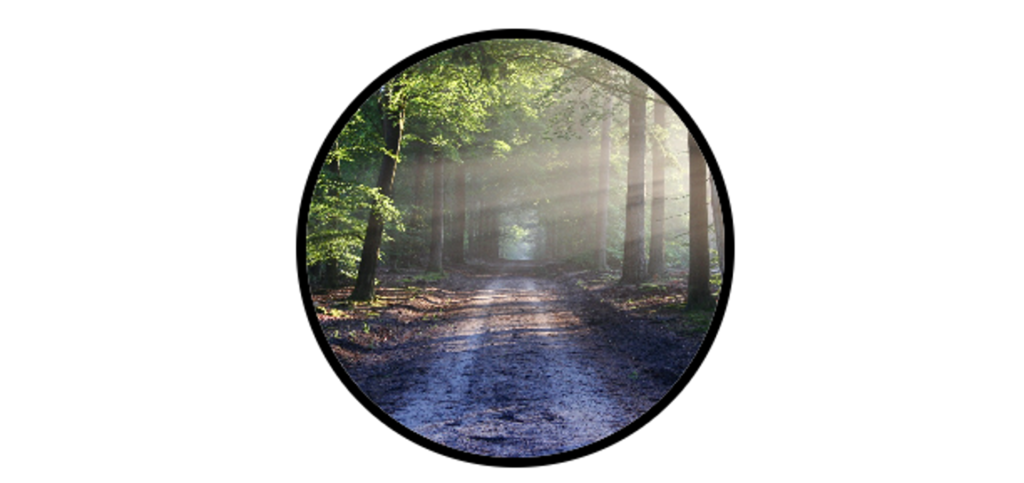
Explanation:
HTML Structure:
- A container div with an IMG tag using the class circular-image makes up the HTML.
- If necessary, the container aids in centering the image both horizontally and vertically.
CSS Styling:
- Flexbox is used by. image-container for centering the image in the viewport.
- Round-image
.> Width and height: Verify that the picture is square, which is required for circular cropping.
.> Border-radius: 50%: Creates a perfect circle in the image by rounding the corners.
.> Object-fit: cover: Assures that the picture fits inside the circle frame at the proper scale.
.> Border: Encircles the picture with a border. The border’s color, width, & style can all be altered as needed.
Full Example:
This is the whole code to create a bordered, circular image.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Circular Image with Border Example</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.circular-image {
width: 250px; /* Set the width */
height: 250px; /* Set the height to the same value as the width */
border-radius: 50%; /* Make the image circular */
object-fit: cover; /* Ensure the image covers the entire area */
border: 5px solid #000; /* Add a black border around the image */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="circular-image">
</div>
</body>
</html>
Put the path of your image file in lieu of your-image.jpg. With a black border, this code will produce a circular image that is centered in the viewport. By changing the border property in the CSS, you can change the border’s color and size.
CSS Image Hover Effects
A more interesting and dynamic user experience can be produced by giving images hover effects. The following are some typical hover effects that you can use CSS to apply to CSS images:
Basic Image Hover Effects
Zoom In on Hover
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Hover Effects</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.hover-image {
width: 300px;
height: auto;
transition: transform 0.3s; /* Smooth transition */
}
.hover-image:hover {
transform: scale(1.1); /* Zoom in on hover */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="hover-image">
</div>
</body>
</html>
Rotate on Hover
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Hover Effects</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.hover-image {
width: 300px;
height: auto;
transition: transform 0.3s; /* Smooth transition */
}
.hover-image:hover {
transform: rotate(15deg); /* Rotate on hover */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="hover-image">
</div>
</body>
</html>
Grayscale on Hover
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Hover Effects</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.hover-image {
width: 300px;
height: auto;
transition: filter 0.3s; /* Smooth transition */
}
.hover-image:hover {
filter: grayscale(100%); /* Grayscale on hover */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="hover-image">
</div>
</body>
</html>
Opacity Change on Hover
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Hover Effects</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.hover-image {
width: 300px;
height: auto;
transition: opacity 0.3s; /* Smooth transition */
}
.hover-image:hover {
opacity: 0.7; /* Change opacity on hover */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="hover-image">
</div>
</body>
</html>
Shadow on Hover
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Hover Effects</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Center the image vertically */
}
.hover-image {
width: 300px;
height: auto;
transition: box-shadow 0.3s; /* Smooth transition */
}
.hover-image:hover {
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.3); /* Add shadow on hover */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="hover-image">
</div>
</body>
</html>
Explanation
Zoom In on Hover:
Transform: scale(1.1): When the image is hovered, it is scaled up by 10%.
Rotate on Hover:
Transform: rotate(15deg): Hovering over the image causes it to rotate by 15 degrees.
Grayscale on Hover:
Filter: grayscale(100%): Hovering over the image applies a grayscale filter.
Opacity Change on Hover:
Opacity: 0.7: Hovering reduces the transparency to 70%.
Shadow on Hover:
Box-shadow: 0 4px 8px rgba(0, 0, 0, 0.3): Hovering over an element adds a shadow effect.
A more interesting and dynamic user experience can be produced by giving images hover effects. The following are some typical hover effects that you can use CSS to apply to images:
Responsive Images
Images that respond Make sure your photos adjust appropriately for a variety of screen sizes & devices. Here’s how to use CSS and HTML to create responsive images:
Using CSS for Responsive Images
Basic Responsive Image
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Image Example</title>
<style>
.responsive-image {
width: 100%;
height: auto; /* Maintain aspect ratio */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="responsive-image">
</div>
</body>
</html>
In this example:
- To ensure that the CSS image scales with the width of the container, its width has been set to 100% of the container.
- To preserve the aspect ratio, the height is left at auto.
Responsive CSS Image with Max-Width
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Image with Max-Width</title>
<style>
.responsive-image {
max-width: 100%;
height: auto; /* Maintain aspect ratio */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="responsive-image">
</div>
</body>
</html>
In this example:
- maximum width: 100% guarantees that the image keeps its original size while scaling down with its container.
Using Picture Element for Responsive CSS Images
More control over the image to display according to screen size, resolution, and various other parameters is available with the element.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Picture Example</title>
<style>
.responsive-image {
width: 100%;
height: auto; /* Maintain aspect ratio */
}
</style>
</head>
<body>
<div class="image-container">
<picture>
<source srcset="image-large.jpg" media="(min-width: 800px)">
<source srcset="image-medium.jpg" media="(min-width: 400px)">
<img src="image-small.jpg" alt="Sample Image" class="responsive-image">
</picture>
</div>
</body>
</html>
In this example:
- Multiple elements that provide various images at different screen sizes are contained in the element.
- In the event that none of the source images match, the element offers a backup CSS image.
Using Media Queries for Responsive
You can apply different CSS rules according on the size of the viewport by using media queries.
CSS Images
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Images with Media Queries</title>
<style>
.responsive-image {
width: 100%;
height: auto; /* Maintain aspect ratio */
}
@media (min-width: 800px) {
.responsive-image {
width: 50%; /* 50% width for larger screens */
}
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="responsive-image">
</div>
</body>
</html>
In this example:
- By default, the image’s width is 100%.
- 50% is the picture width for screens 800 pixels wide and wider.
Combining Techniques
These methods can be combined to produce responsive designs that are more flexible and intricate.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Advanced Responsive Image Example</title>
<style>
.image-container {
display: flex;
justify-content: center;
align-items: center;
padding: 20px;
}
.responsive-image {
max-width: 100%;
height: auto; /* Maintain aspect ratio */
border: 2px solid #ccc; /* Optional: Add a border */
border-radius: 10px; /* Optional: Add rounded corners */
}
</style>
</head>
<body>
<div class="image-container">
<picture>
<source srcset="image-large.jpg" media="(min-width: 800px)">
<source srcset="image-medium.jpg" media="(min-width: 400px)">
<img src="image-small.jpg" alt="Sample Image" class="responsive-image">
</picture>
</div>
</body>
</html>
In this example:
- The picture adapts to the size of its container.
- Depending on the screen size, several image sources are used.
- For a better look, more styling is added, such as borders & rounded edges.
You can make sure that your photos appear fantastic on every device & screen size by employing these approaches.
Applying Filters
Using CSS to apply filters to photos, one may achieve a variety of visual effects, including blur, sepia, grayscale, and more. Here’s how to use CSS to add various filters to CSS images:
Basic Filters
Grayscale Filter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Grayscale Filter</title>
<style>
.grayscale-image {
width: 300px;
height: auto;
filter: grayscale(100%); /* Apply grayscale filter */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="grayscale-image">
</div>
</body>
</html>
Sepia Filter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sepia Filter</title>
<style>
.sepia-image {
width: 300px;
height: auto;
filter: sepia(100%); /* Apply sepia filter */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="sepia-image">
</div>
</body>
</html>
Blur Filter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Blur Filter</title>
<style>
.blur-image {
width: 300px;
height: auto;
filter: blur(5px); /* Apply blur filter */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="blur-image">
</div>
</body>
</html>
Brightness Filter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Brightness Filter</title>
<style>
.brightness-image {
width: 300px;
height: auto;
filter: brightness(150%); /* Apply brightness filter */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="brightness-image">
</div>
</body>
</html>
Contrast Filter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contrast Filter</title>
<style>
.contrast-image {
width: 300px;
height: auto;
filter: contrast(200%); /* Apply contrast filter */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="contrast-image">
</div>
</body>
</html>
Combining Multiple Filters
Several filters can be combined to create a distinctive effect. For instance, blending sepia and grayscale:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Combined Filters</title>
<style>
.combined-filters-image {
width: 300px;
height: auto;
filter: grayscale(50%) sepia(50%); /* Apply multiple filters */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="combined-filters-image">
</div>
</body>
</html>
Hover Effects with Filters
Additionally, you can apply filters while hovering to produce interactive effects:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hover Effects with Filters</title>
<style>
.hover-filter-image {
width: 300px;
height: auto;
transition: filter 0.3s; /* Smooth transition */
}
.hover-filter-image:hover {
filter: grayscale(100%) blur(2px); /* Apply filters on hover */
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Sample Image" class="hover-filter-image">
</div>
</body>
</html>
In this example:
- transition: a smooth transition is produced by filtering for 0.3 seconds
- When an image is hovered over, the filters grayscale(100%) and blur(2px) are applied.
Full Example with Various Filters
This is a comprehensive example that incorporates multiple hover effects and filters:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Filters Example</title>
<style>
.image-container {
display: flex;
justify-content: space-around;
flex-wrap: wrap;
gap: 20px;
padding: 20px;
}
.filter-image {
width: 300px;
height: auto;
transition: filter 0.3s; /* Smooth transition */
}
.filter-image.grayscale:hover {
filter: grayscale(100%);
}
.filter-image.sepia:hover {
filter: sepia(100%);
}
.filter-image.blur:hover {
filter: blur(5px);
}
.filter-image.brightness:hover {
filter: brightness(150%);
}
.filter-image.contrast:hover {
filter: contrast(200%);
}
.filter-image.combined:hover {
filter: grayscale(50%) sepia(50%);
}
</style>
</head>
<body>
<div class="image-container">
<img src="your-image.jpg" alt="Grayscale Image" class="filter-image grayscale">
<img src="your-image.jpg" alt="Sepia Image" class="filter-image sepia">
<img src="your-image.jpg" alt="Blur Image" class="filter-image blur">
<img src="your-image.jpg" alt="Brightness Image" class="filter-image brightness">
<img src="your-image.jpg" alt="Contrast Image" class="filter-image contrast">
<img src="your-image.jpg" alt="Combined Filters Image" class="filter-image combined">
</div>
</body>
</html>
In this example:
- A unique hover filter is applied to every image.
- The images are arranged nicely with space surrounding them in the container thanks to Flexbox.
These illustrations show you how to apply CSS filters to improve an image’s visual appeal and produce eye-catching effects for viewers.
Background Images
You can apply images to components like or as other containers through the use of background images in CSS. Here’s how to change the properties of background images and set them:
Basic Background CSS Image
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Background Image Example</title>
<style>
.container {
width: 100%;
height: 400px;
background-image: url('your-image.jpg');
background-size: cover; /* Cover the entire container */
background-position: center; /* Center the image */
background-repeat: no-repeat; /* Do not repeat the image */
}
</style>
</head>
<body>
<div class="container">
<!-- Content inside the container -->
</div>
</body>
</html>
Explanation:
- container: This CSS class is applied to the
- element, which is going to be our background image’s container.
- background-image: Indicates the picture’s URL to be used as the backdrop.
- background-size: Defines the dimensions of the background picture. cover guarantees that the picture fills the container without squishing or stretching.
- background-position: Specifies the location of the background CSS image inside its container. The image is centered both vertically and horizontally in the center.
- background-repeat: Indicates if you want the background CSS image to repeat (no-repeat indicates that it won’t).
Responsive Background Image
As demonstrated in the example above, you may use the background-size: cover; property to make the background picture responsive, meaning it will change to fit different screen sizes. By doing this, the background image is guaranteed to scale properly to fill the container.
Fixed Background Image
Use background-attachment: fixed; : if you want a background CSS image to remain fixed as the rest of the content scrolls.
.container {
background-image: url('your-image.jpg');
background-size: cover;
background-position: center;
background-repeat: no-repeat;
background-attachment: fixed; /* Keeps the background fixed */
}
Gradient Overlay on Background Image
For an even more stylish look, you can place a gradient overlay over the background CSS image:
.container {
position: relative; /* Ensure the container is positioned relative */
background-image: url('your-image.jpg'), linear-gradient(to bottom right, rgba(0,0,0,0.5), rgba(0,0,0,0)); /* Overlay gradient */
background-size: cover;
background-position: center;
background-repeat: no-repeat;
height: 400px;
}
.container::before {
content: ''; /* Ensure pseudo-element is empty */
position: absolute; /* Position absolutely within its container */
top: 0;
left: 0;
width: 100%;
height: 100%;
background: linear-gradient(to bottom right, rgba(0,0,0,0.5), rgba(0,0,0,0)); /* Gradient overlay */
}
In this example:
- Background-CSS image has a gradient (linear-gradient(…)) and an image URL (URL (‘your-image.jpg’)) for the overlay effect.
- Before the pseudo-element is used to generate the gradient overlay, placed exactly inside the. container.
Full Example
Here’s a comprehensive example that combines different backdrop image techniques:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Background Image Example</title>
<style>
.container {
width: 100%;
height: 400px;
background-image: url('your-image.jpg');
background-size: cover;
background-position: center;
background-repeat: no-repeat;
position: relative;
}
.overlay {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: linear-gradient(to bottom right, rgba(0,0,0,0.5), rgba(0,0,0,0));
}
</style>
</head>
<body>
<div class="container">
<div class="overlay"></div>
<!-- Content inside the container -->
</div>
</body>
</html>
Summary
- Background- CSS image: Configures the image’s URL.
- Background-size: Specifies the size (cover, contain, etc.) of the CSS image.
- Background-position: Indicates the location of the background CSS image inside its container.
- Backdrop-repeat: Regulates whether and how the image in the backdrop is repeated.
- Backdrop-attachment: Controls whether the backdrop picture moves with the text or remains still.
- Linear-gradient: Overlays a gradient on top of the image in the backdrop.
You may make background images for your web pages that are both aesthetically pleasing and responsive by using these strategies. Adapt the properties to your own design requirements.
Summary
These illustrations show off different CSS image manipulation and styling techniques. You can combine these methods to get the desired results for your website design.