Table of Contents
ToggleCSS Selectors
The foundation of any well-designed webpage is the CSS selector. They let you add styles depending on the id, class, type, attribute, and more of the HTML elements on your website. This tutorial will explore the particulars of CSS selectors and how important they are to improving the look and feel of your web pages.
Types of CSS Selectors
There are several varieties of CSS selectors, and each selects HTML elements differently. Let’s investigate them:
CSS Selectors | Description |
Simple Selector | It is employed to choose HTML elements according to their attributes, id, element name, etc. |
Universal Selector | chooses every element on the page. |
Group Selector | Group selectors are used to apply the same styles to multiple selectors at once by separating them with commas. |
Attribute Selector | Targets elements according to the values of their attributes. |
Pseudo-Class Selector | It uses selectors, like hover for hover effects, to pick items according to their position or state. |
Pseudo-Element Selector | Picks out particular elements, such:: before or after. |
Simple Selectors
The classes below are contained in simple selectors.
Simple Selectors | Description |
Element Selector | Uses the tag names of HTML elements to choose them. |
Id Selector | It targets an HTML element that has a particular id property. |
Class Selector | Identify elements that possess a specific class attribute. |
Example: To better understand selectors and their applications, we will develop the code in this example.
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<title>CSS Selectors</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1>Sample Heading</h1>
<p>This is Content inside first paragraph</p>
<div id="div-container">
This is a div with id div-container
</div>
<p class="paragraph-class">
This passage has a class-paragraph structure.
</p>
</body>
</html>
Note: The example above will be subject to CSS regulations.
Element Selector
HTML elements are chosen by the element selector according to their element name (or tag), such as p, h1, div, span, etc.
NOTE: The example above uses the following code. All <p> and <h1> elements had the CSS rules implemented, as you can see.
CSS:
body{
background-color: aliceblue;
}
h1 {
color: red;
font-size: 3rem;
}
p {
color: white;
background-color: gray;
}
Output:
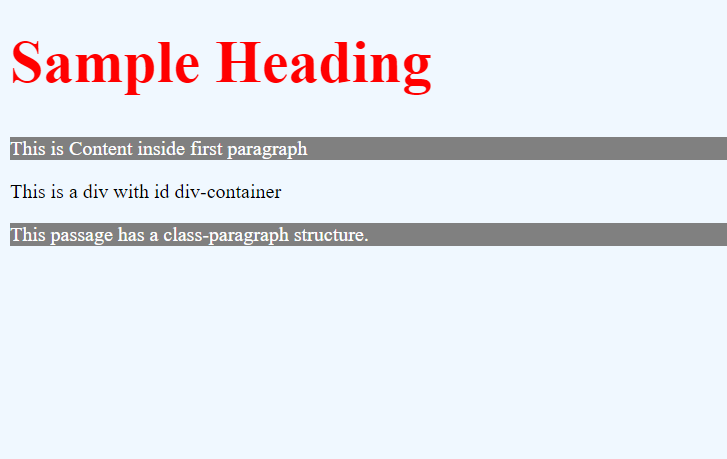
Id Selector
The id selector selects a particular HTML element by using its id attribute. To utilize the id selector, an element’s id must be unique on the page.
Note: The id selector is used in the following code in the example above.
CSS:
body{
background-color: aliceblue;
}
#div-container{
color: blue;
background-color: gray;
}
Output:
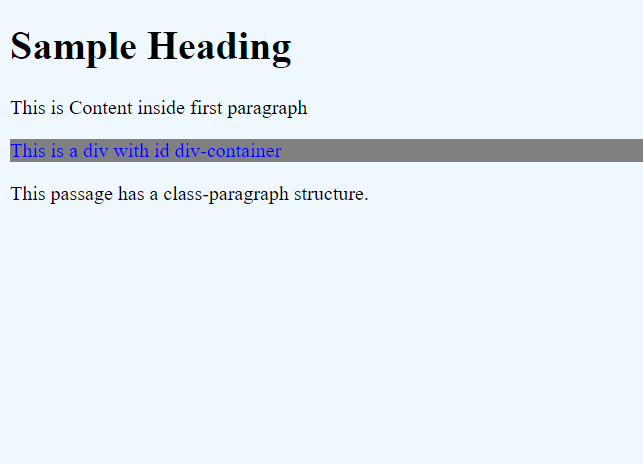
Class Selector
HTML elements having a certain class attribute are chosen by the class selector.
Note: The class selector is used in the following code in the example above. In CSS, you have to use (. ) followed by the class name to use a class selector. The HTML element with the class attribute “paragraph-class” will be subject to this rule.
CSS:
.paragraph-class {
color: white;
font-family: monospace;
background-color: purple;
}
Output:
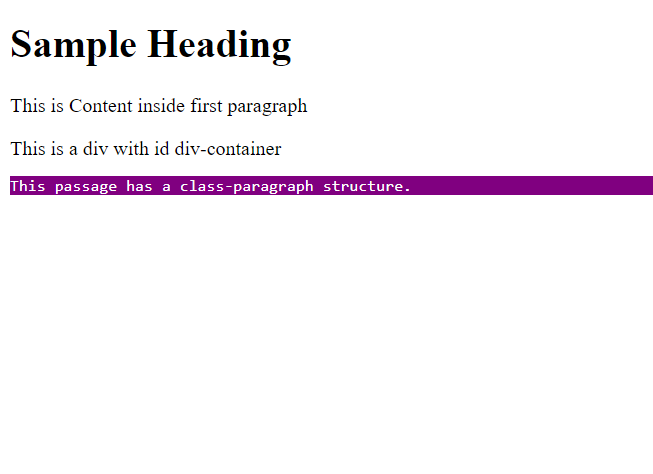
Universal Selector
In CSS, all items within an HTML document can be selected with the Universal selector (*). It also contains additional elements that are included within another element.
Note: The universal selector in the example above is used with the following code. Every single HTML element on the page will be subject to the following CSS rule:
CSS:
* {
color: white;
background-color: black;
}
Output:
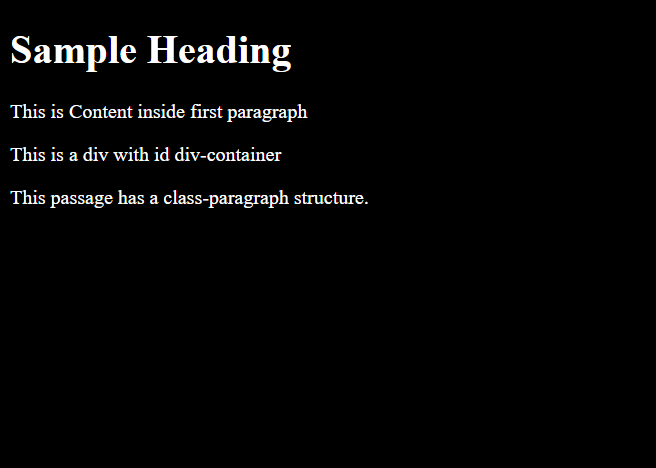
Group Selector
To apply the same style to all comma-separated components, use the Group selector.
Note: If you would like to apply common styles to many selectors, you can put the rules in groups as indicated below, rather than individually.
CSS:
#div-container, .paragraph-class, h1{
color: white;
background-color: purple;
font-family: monospace;
}
Output:
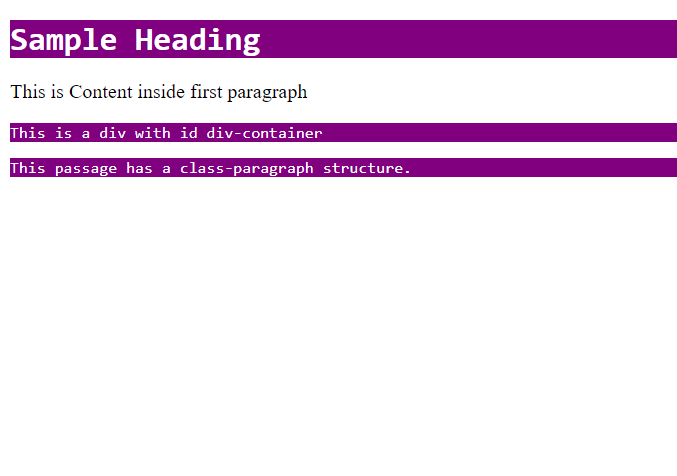
Enhance your proficiency in web design with our collection of HTML and CSS guides and references.
Attribute Selector
An attribute selector in CSS allows you to target HTML elements based on the presence or value of their attributes. It enables you to apply styles to elements that have specific attributes or attribute values.
Here’s a breakdown of how attribute selectors work:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<title>CSS Selectors</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1>Sample Heading</h1>
<p>This is Content inside first paragraph</p>
<div id="div-container">
This is a div with id div-container
</div>
<p class="paragraph-class">
This passage has a class-paragraph structure.
</p>
<a href="https://example.com">Learn HTML</a>
<a href="https://example.com">Learn CSS</a>
<a href="https://example.com">Learn JavaScript</a>
</body>
</html>
CSS:
[href] {
background-color: lightgreen;
color: black;
font-family: monospace;
font-size: 1rem;
}
Output:
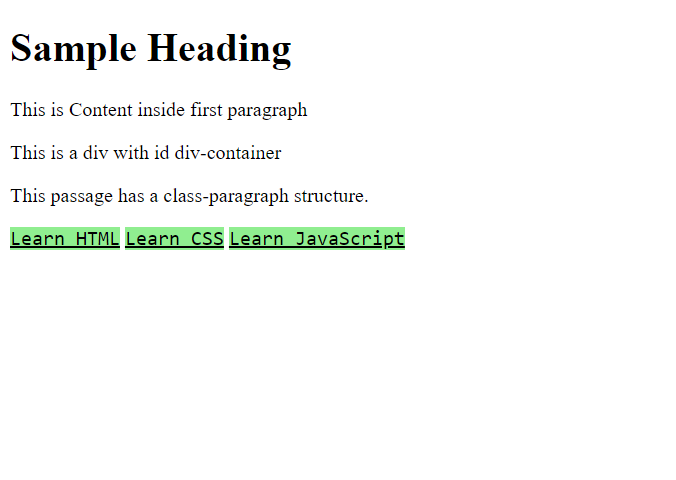
Pseudo-Class Selector
It can be used to style a certain kind of elemental state. As an illustration, it’s employed to style an element while the mouse pointer is over it.
Note: When dealing with a pseudo-class selector, we utilize a single colon (:).
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<title>CSS Selectors</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1>Sample Heading</h1>
<p>This is Content inside first paragraph</p>
<div id="div-container">
This is a div with id div-container
</div>
<p class="paragraph-class">
This passage has a class-paragraph structure.
</p>
<a href="https://example.com">Learn HTML</a>
<a href="https://example.com">Learn CSS</a>
<a href="https://example.com">Learn JavaScript</a>
</body>
</html>
CSS:
h1:hover{
background-color: aqua;
}
Output:
Pseudo-Element Selector
It is employed to style any particular segment of the component. For instance, the first letter or line of any element can be styled using this styling method.
Note: In the case of a pseudo-element selector, we employ a double colon (::).
Syntax:
Selector: Pseudo-Element{
Property: Value;
}
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<title>CSS Selectors</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1>Sample Heading</h1>
<p>This is Content inside first paragraph</p>
<div id="div-container">
This is a div with id div-container
</div>
<p class="paragraph-class">
This passage has a class-paragraph structure.
</p>
<a href="https://example.com">Learn HTML</a>
<a href="https://example.com">Learn CSS</a>
<a href="https://example.com">Learn JavaScript</a>
</body>
</html>
CSS:
p::first-line{
background-color: goldenrod;
}
Output:
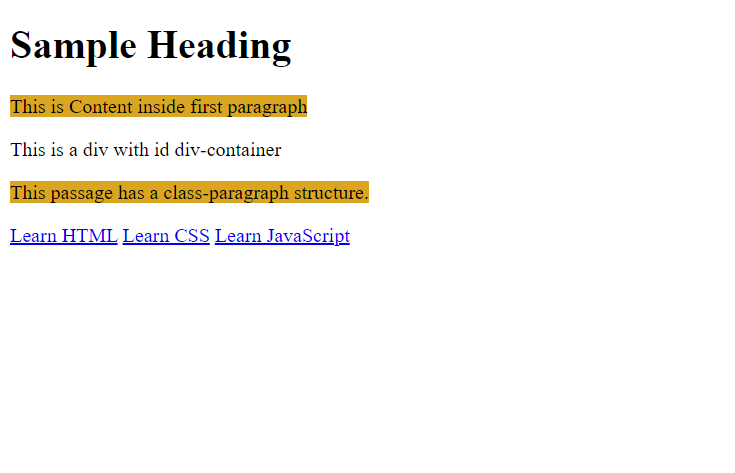
In conclusion, mastering CSS selectors opens up a world of possibilities for web designers and developers. By understanding how to target and style elements effectively, you can create visually stunning and responsive websites that engage users across various devices. Whether you’re a novice or a seasoned professional, continued practice and exploration of CSS selectors will enhance your skill set and enable you to craft exceptional web experiences. So, dive in, experiment, and unleash the full potential of CSS selectors in your next project!