Are you new to web development and looking for a technology that is easy to learn and has a large community to support you? Look no further than ReactJS! ReactJS is a JavaScript library that is widely used in the web development world due to its ease of use, high performance, and strong community support. In this beginner’s guide, we will cover everything you need to know to get started with React web development in 2023.
Table of Contents
ToggleWhat is React?
User interfaces can be created using the JavaScript library React. It was created by Facebook and is now maintained by Facebook and a large community of developers. React allows developers to create complex user interfaces with reusable components, making the process of building web applications more efficient and scalable.

Why choose to React for web development?
There are several reasons why developers choose React for web development:
- Ease of use: React is easy to learn and use, especially for developers who are already familiar with JavaScript.
- High performance: React’s virtual DOM allows for fast rendering of web pages, resulting in a smoother user experience.
- Reusable components: React’s component-based architecture allows designers to create reusable components, creating the development process more efficient and scalable.
- Strong community support: React has a large and active community of developers who contribute to its development and offer support through forums, documentation, and tutorials.
Read also:- Best Ways To Learn Python Programming For Beginners In 2023
Installing React
Before you can start building a React app, you need to install React on your system. You can install React using Node.js and npm (Node Package Manager), which is a tool for managing JavaScript packages.
To install React, follow these steps:
- Install Node.js and npm on your system.
- Open a terminal window and navigate to the directory where you want to create your React app.
- Run the following command to create a new React app:
npx create-react-app my-react
This will create a new React app in a directory called “my-react.”
Creating your first React app
Once you have installed React, you can create your first React app. Follow these steps to create a basic React app:
- Open a terminal window and navigate to the directory where you want to create your React app.
- Run the following command to create a new React app:
npx create-react-app my-react
- Navigate to the newly created directory:
cd my-react
- Start the development server:
npm start
Your React app will launch in a new browser window as a result.
Components in React
A component in React is a reusable chunk of code that simulates a particular element of a user interface. Components can either be class-based or functional. Functional components are simpler and easier to write, while class-based components offer more functionality.
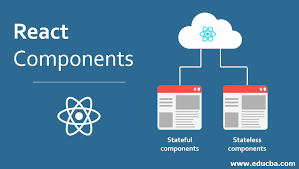
To create a functional component in React, follow these steps:
- Create a new file called “MyComponent.js” in the “src” directory.
- Add the following code to the file:
import React from 'react';
function MyComponent(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default MyComponent;
- After creating a functional component in React, you can use it in other parts of your application by importing it and including it in your JSX code. For example, if you want to use the MyComponent function in a different file, you can import it using the following code:
- Then, in your JSX code, you can use the component like this:
- This will render the component with the name “John” passed in as a prop. You can pass any other props to the component as needed.
- Additionally, class-based components can be created by extending the React.Component class and defining a render method. These components offer more advanced features like state and lifecycle methods.
Props and state in React
Props and state are two essential concepts in React. Props are utilized to pass data from a parent component to a child component, while the state is used to collect data within a component.
To pass props from a parent component to a child component, you can use the following syntax:
<ChildComponent propName={propValue} />
To use props in a child component, you can access them through the props
object, like this:
function ChildComponent(props) {
return <h1>Hello, {props.propName}!</h1>;
}
State, on the other hand, is used to manage data within a component. To use state in a class-based component, you need to define a state object in the component’s constructor, like this:
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
render() {
return <h1>Count: {this.state.count}</h1>;
}
}
You can then update the state using the setState()
method, like this:
this.setState({ count: this.state.count + 1 });
JSX in React
JSX is a syntax extension for JavaScript that permits you to write HTML-like code in your JavaScript files. JSX creates it easier to write and read code in React.
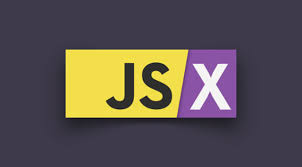
Here’s an example of JSX code:
function MyComponent(props) {
return (
<div>
<h1>Hello, {props.name}!</h1>
<p>Welcome to my React app.</p>
</div>
);
}
Handling events in React
In React, you can handle events like you would in HTML using the onClick
attribute. Here’s an example:
function MyComponent(props) {
function handleClick() {
console.log('Button clicked.');
}
return (
<button onClick={handleClick}>Click me</button>
);
}
Lifecycle methods in React
Lifecycle methods are special methods that are called at different stages of a component’s life cycle. These methods can be used to perform certain actions when a component is mounted, updated, or unmounted.
Here are a few typical React lifecycle methods:
componentDidMount
: Called after the component is mounted.componentDidUpdate
: Called after the component is updated.componentWillUnmount
: just before the component is unmounted.
React Router
A well-liked library for managing routing in React apps is called React Router. It allows you to define routes and map them to different components.
Here’s an example of how to use React Router:
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function Home() {
return <h1>Welcome to my app!</h1>;
}
function About() {
return <h1>About us</h1>;
}
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
</Router>
);
}
Redux in React
A well-liked state-management library for React apps is Redux. It allows you to manage the state of your app in a centralized location, making it easier to maintain and debug your code.
To use Redux in your React app, you need to install the redux
and react-redux
libraries. Here’s a sample of how to use Redux in a React component:
import React from 'react';
import { connect } from 'react-redux';
class MyComponent extends React.Component {
render() {
return (
<div>
<h1>Count: {this.props.count}</h1>
<button onClick={this.props.increment}>Increment</button>
<button onClick={this.props.decrement}>Decrement</button>
</div>
);
}
}
function mapStateToProps(state) {
return {
count: state.count,
};
}
function mapDispatchToProps(dispatch) {
return {
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' }),
};
}
export default connect(mapStateToProps, mapDispatchToProps)(MyComponent);
In this example, the mapStateToProps
function maps the Redux state to the component’s props, and the mapDispatchToProps
function maps the Redux actions to the component’s props. The connect
function connects the component to the Redux store.
React Hooks
React Hooks are a feature introduced in React 16.8 that allows you to use state and additional React features in functional components. Hooks provide a simpler and more concise way to write React code.
Here’s an example of how to use the useState
hook to manage state in a functional component:
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return (
<div>
<h1>Count: {count}</h1>
<button onClick={handleClick}>Click me</button>
</div>
);
}
Conclusion
In this beginner’s guide to React web development, we’ve covered the basics of React, including components, props, state, JSX, handling events, lifecycle methods, React Router, Redux, and React Hooks. While there’s still much to learn, this guide should give you a solid foundation to build upon.
Read also:- Choosing The Right Computer Science Degree: Tips And Recommendations From Reddit
FAQs
What is React?
User interfaces can be created using the JavaScript library React.
Do I need to learn JavaScript before learning React?
Yes, you need to have a good understanding of JavaScript before learning React.
What is JSX?
You can write HTML-like code in your JavaScript files through to the JSX syntax extension.
What is Redux?
A well-liked state management library for React apps is Redux.
What are React Hooks?
State and other React features can be used in functional components due to the React Hooks feature, which was added in React 16.8.